Build your own HTTPS server with Delphi – Open SSL Example
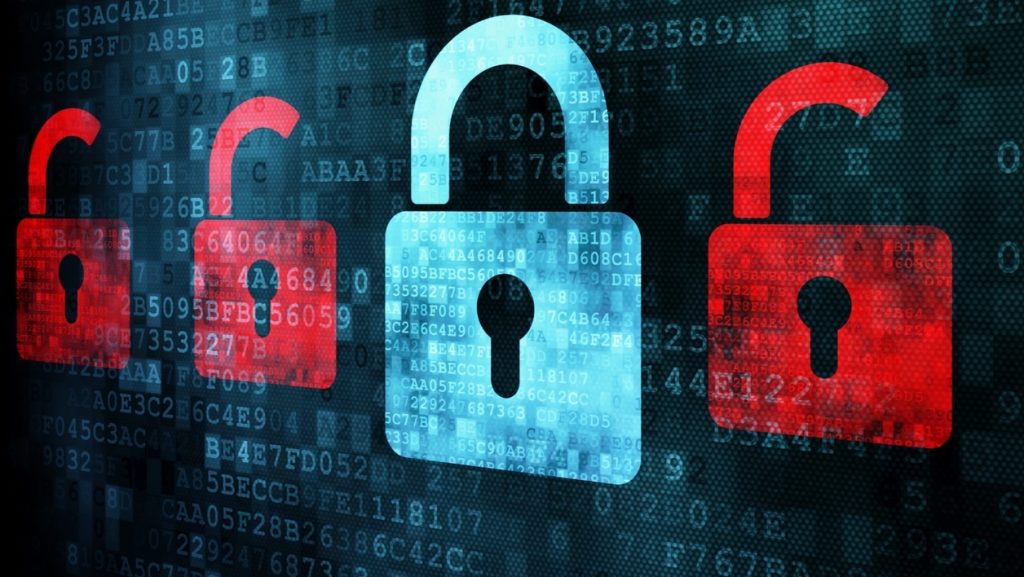
Assuming that you know how to make a web server with TidHttpServer, but now you need to put your server in HTTPS, so to do that you need to add to your application the TIdServerIOHandlerSSLOpenSSL and set it as the IOHandler of your TidHTTpServer.
Now you need a valid and unique certificate, to make some tests you can use an Open SSL certificate, to have it you can write this command, and compile all question that openssl do, don’t forget the password you specified:
ivan@HARDTERRON5:~$ openssl req -x509 -nodes -days 365 -newkey rsa:1024 -keyout mycert.pem -out mycert.pem
this certificate is self signed, so when you use a browser to view your server pages the browser make a security information to you. We normally buy a Wildcard certificate for our server on Netsons.com and create a private key with apache.
After that we put the key and the certificate in the same file:
-----BEGIN PRIVATE KEY----- MIICdwIBADANBgkqhkiG9w0BAQEFAASCAmEwggJdAgEAAoGBAMKsM2fFtB2+6gwJ qx5crCF2noge5ZDx1V5mCWHBH82wzEa3DEJ/HJjMl6yOJ56yVOsPophx7IPzS7/N ... sQL72X40rjFuFrnWrepnVmbzojMsBQJABTxe4vrQxlXDQE202WFSB34bRph+IQuT bhWtii0MLiifaw6611f2DITS2Qq5XdkKRExymuNR6+yHfXFjSOhj/wJBAJcGcYuH Rme1eHoRaofgajFwzxKbkAXnZjx7JSttDiZWLDpZXbbQdvK/i38JOg7zvmjXUNSH tnvpoafBLbVhHQo= -----END PRIVATE KEY----- -----BEGIN CERTIFICATE----- MIIC7DCCAlWgAwIBAgIJAPIOReOspuhaMA0GCSqGSIb3DQEBCwUAMIGOMQswCQYD VQQGEwJJVDEOMAwGA1UECAwFSVRBTFkxDzANBgNVBAcMBk1JTEFOTzESMBAGA1UE CgwJU1lOQVBUSUNBMRIwEAYDVQQLDAlMQVRUQU5aSU8xDzANBgNVBAMMBlJFTU9E RTElMCMGCSqGSIb3DQEJARYWaXZhbi5yZXZlbGxpQGdtYWlsLmNvbTAeFw0xNjA5 MjAxMDI4NDJaFw0xNzA5MjAxMDI4NDJaMIGOMQswCQYDVQQGEwJJVDEOMAwGA1UE ... rI4nnrJU6w+imHHsg/NLv82GmvinogMvYSiLcYPHVeof0wfIPfCOYTihhwPebqBP YlWaG18R3mzxJrUBewjOTyY4hZxduRAzLuCCWCEBmom8pe/iPhUCAwEAAaNQME4w HQYDVR0OBBYEFCT6EDaCYxwdJQmd7Fy2PO/JYbRbMB8GA1UdIwQYMBaAFCT6EDaC YxwdJQmd7Fy2PO/JYbRbMAwGA1UdEwQFMAMBAf8wDQYJKoZIhvcNAQELBQADgYEA KkoIL/FRhbEDVlWmYVxc8jVpXYZ0pM37PCEP23qyCnyJkTmTWsS8NxfPNUlXuX/j +ZrUxo7n4Y+gm9SkDNWHxG5kMG0cun+OJUb6HzkuIWlzYplXveMb0vLpBWmhKhxK YHysyIBL6UBxfZqxn2KHlIna+8uUvnvSVd9aHJhQlDs= -----END CERTIFICATE-----
Now we have “mycert.pem” file, and we need to put that in the application folder, and we can configure the TIdServerIOHandlerSSLOpenSSL to manager the certificate like this:
after this you need to manage two events on the TIdServerIOHandlerSSLOpenSSL like this:
function TForm1.IdServerIOHandlerSSLOpenSSL1VerifyPeer(Certificate: TIdX509;
AOk: Boolean; ADepth, AError: Integer): Boolean;
begin
if ADepth = 0 then
begin
Result := AOk;
end
else
begin
Result := True;
end;
end;
procedure TForm1.IdServerIOHandlerSSLOpenSSL1GetPassword(var Password: string);
begin
Password := '';
end;
To run the application, for example on a Win32 system you need to add the openSSL libraries to you appication folder:
to obtain (download) the newest release of openssl library you can visit : https://indy.fulgan.com/SSL/
At the end when you buy the certificate for the application the result is:
On newer Delphi version you need to implement “OnQuerySSLPort” method to allow SSL connections on port different to 443 like the next sample code:
type TSSLHelper = class // This helper class is neccessary to set ssl true // as it defaults to false on non standard ssl ports procedure QuerySSLPort(APort: Word; var VUseSSL: boolean); end; TForm16 = class(TForm) IdHTTPServer1: TIdHTTPServer; IdServerIOHandlerSSLOpenSSL1: TIdServerIOHandlerSSLOpenSSL; swOn: TSwitch; IdConnectionIntercept1: TIdConnectionIntercept; IdConnectionIntercept2: TIdConnectionIntercept; procedure swOnSwitch(Sender: TObject); procedure IdHTTPServer1CommandGet(AContext: TIdContext; ARequestInfo: TIdHTTPRequestInfo; AResponseInfo: TIdHTTPResponseInfo); procedure FormCreate(Sender: TObject); private { Private declarations } public SSLHelper : TSSLHelper; { Public declarations } end; var Form16: TForm16; implementation {$R *.fmx} procedure TForm16.FormCreate(Sender: TObject); begin SSLHelper := TSSLHelper.Create; IdHTTPServer1.OnQuerySSLPort := SSLHelper.QuerySSLPort; end; procedure TForm16.IdHTTPServer1CommandGet(AContext: TIdContext; ARequestInfo: TIdHTTPRequestInfo; AResponseInfo: TIdHTTPResponseInfo); begin AResponseInfo.ContentText := 'ciao'; end; procedure TForm16.swOnSwitch(Sender: TObject); begin IdHTTPServer1.Active := swOn.IsChecked; end; { TSSLHelper } procedure TSSLHelper.QuerySSLPort(APort: Word; var VUseSSL: boolean); begin VUseSSL := True; end; end.